Tech & AI
Creating a Personal AI Assistant with GPT and Flask
Building a personal AI assistant is easier than ever with tools like GPT models and Flask. In this post, we will walk you through creating a web-based assistant that can understand and respond to user inputs in a conversational manner.
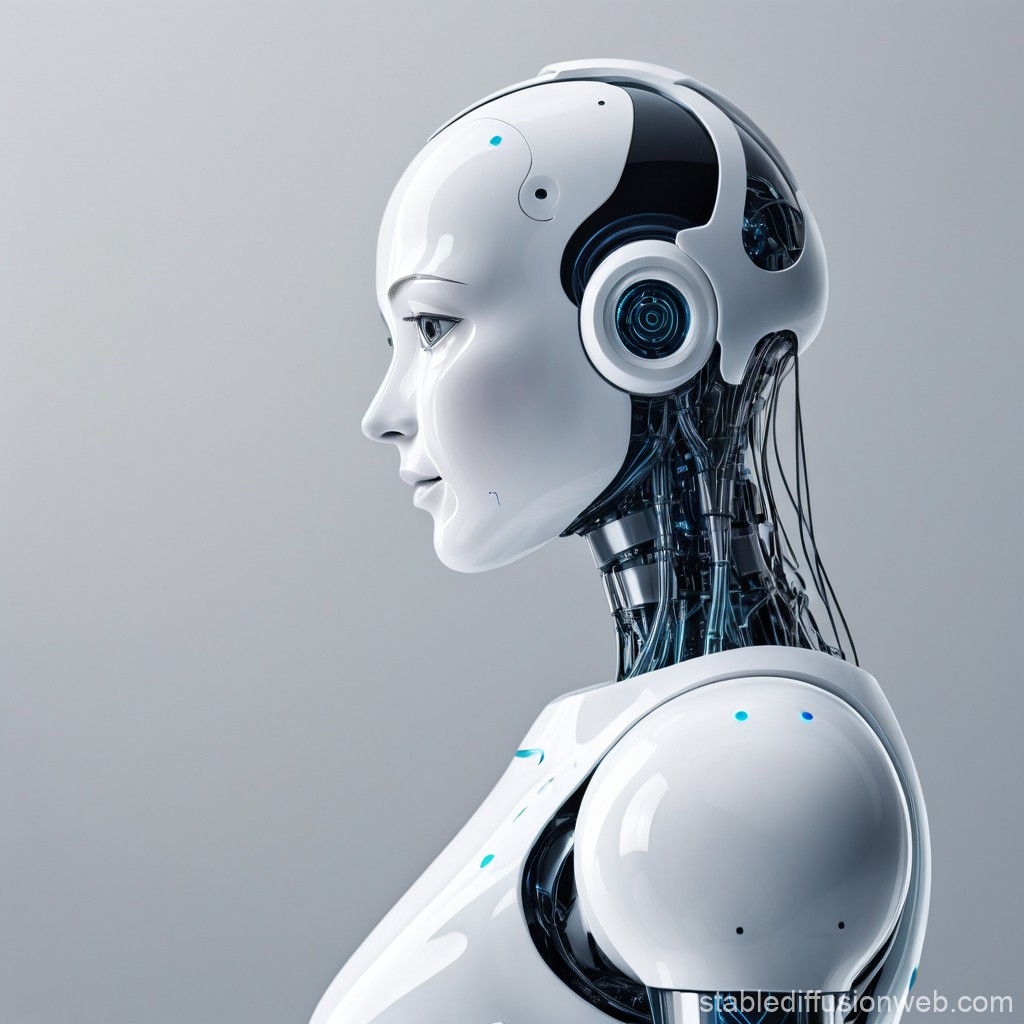
Step 1: Setting Up Your Environment
To start, ensure you have Python installed and set up a virtual environment. You will need Flask for the web framework and OpenAI's GPT API to handle natural language processing.
pip install flask openai
Step 2: Creating the Flask App
Create a new Python file and set up a basic Flask server:
from flask import Flask, request, render_template
import openai
app = Flask(__name__)
@app.route("/", methods=["GET", "POST"])
def index():
if request.method == "POST":
user_input = request.form["input"]
response = get_gpt_response(user_input)
return render_template("index.html", response=response)
return render_template("index.html")
if __name__ == "__main__":
app.run(debug=True)
Step 3: Connecting to GPT
Next, set up your function to interact with the GPT API. You will need your API key from OpenAI:
import openai
def get_gpt_response(prompt):
openai.api_key = "YOUR_API_KEY"
response = openai.Completion.create(
engine="text-davinci-003",
prompt=prompt,
max_tokens=150
)
return response["choices"][0]["text"].strip()
"With just a few lines of code, you can have a fully functional AI assistant running on your local server."
Key Features of the AI Assistant:
-
Understands natural language queries
-
Provides informative responses in real-time
-
Customizable to suit various use cases
Final Thoughts
By following this guide, you can create a personal AI assistant that leverages the power of GPT and Flask. The possibilities are endless, from chatbots to virtual tutors and more.